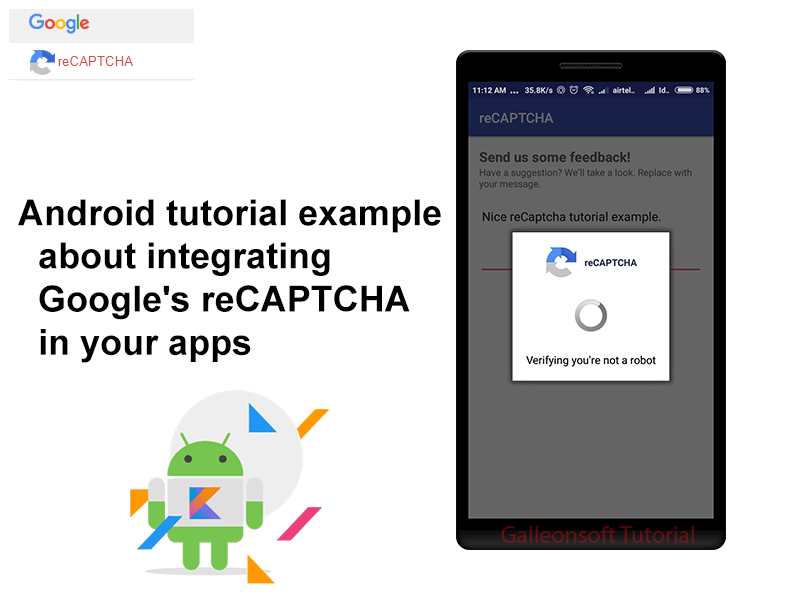
Android example for integrating captcha in android example
May 28, 2018Hello fellas, In this article, we came up with Kotlin JSON Parser Example for Android. As we got many requests about Kotlin JSON parser tutorial, we finally get time to post it for our readers.
Introducing to JSON
JavaScript Object Notation is based on a subset of the JavaScript Programming Language, Standard ECMA-262 3rd Edition – December 1999. JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the programming languages of many others. These properties make JSON an ideal data-interchange language.
Following are some useful facts about JSON:
- JSON full form is JavaScript Object Notation (JSON).
- JSON is a lightweight data-interchange format.
- JSON is very easy for humans to read and write for rest-API or data send and receive.
- JSON is simple for machines to parse and generate machine code.
- The official JSON filenames use the extension .json and media type for JSON is application/JSON.
- The JSON Type code is TEXT.
Kotlin JSON Parser Example for Android
JSON data is built on two structures like object{}, array[] A collection of name/value pairs realized as an object record JSON Object example: In this example name = full_name and value = Jigar Patel
{ "full_name": "Jigar Patel" }
An ordered list of object{} values means array[] JSON Array example: In this example first object = { “full_name” : “Jigar Patel” }
[{ "full_name": "Jigar Patel" }, { "full_name": "Ravi Patel" }]
JSON Parsing in android Kotlin example tutorial for learning and how to parse JSON in Kotlin android using simple and easiest ways. What is the difference between {} and [] (Square brackets and Curly brackets)? The JSON data (node) will start and end with a curly bracket or square bracket. If JSON data is started with { and end with } means it’s JSON object and if JSON data is started with [ and end with ] means it’s JSON array. The JSON value can be a string in double quotes (” “), or a number, or true or false or null, or an object or an array. These structures can be nested on the collection of key/value pairs.
1. Create New Android Project
• Click on Android Studio Icon ( Open Android Studio ).
○ If you have already opened Android Studio from File ⇒ New Project.
• Start a new Android Studio Project. Then fill the displayed form.
○ See below-required details like this with an example.
○ Application name (Example: My AppName),
○ Company domain (Example: galleonsoft.com),
○ Project location (Example: C:\Users\JIGARPATEL\Desktop\Projects\MyAppName),
○ Package name (Example: com.galleonsoft.myappname),
○ Check Include Kotlin Support.
• Select the form factors and minimum SDK
○ Check Phone and Tablet.
• Add an Activity to Mobile you should Select Empty Activity.
○ You can select Basic Activity / Empty Activity. More options are available.
• Create a new empty activity.
○ Enter Activity Name (Example: MainActivity).
○ Enter Layout Name (Example: activity_main).
• Finish
○ Wait for few minutes to creating and building your projects.
2. Set XML Layout (UI)
Open the layout file of ( activity_jsonparser_kotlin.xml ) and add the below XML code.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <ProgressBar android:id="@+id/Pbar" android:layout_width="match_parent" android:layout_height="100dp" android:layout_gravity="center" android:visibility="gone" /> <ListView android:id="@+id/Lv_developers" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="10dp" android:dividerHeight="12dp" /> </LinearLayout>
3. Create the layout file for listview item row ( simple_listview_item.xml ) and add the below XML code.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="16dp" android:orientation="vertical"> <TextView android:id="@+id/tv_name" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="4dp" android:textColor="@color/colorPrimaryDark" android:textSize="16sp" android:textStyle="bold" /> <TextView android:id="@+id/tv_age" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="4dp" android:textColor="@color/colorPrimary" /> <TextView android:id="@+id/tv_city" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="4dp" android:textColor="@color/colorAccent" /> </LinearLayout>
4. Set Kotlin Android Code
Create a new class named ApiGetPostHelper.kt This Object/Class GET And POST API. Also, it’s helps in others projects. This class required for HttpURLConnection Method. You can use alternatively Volley, Retrofit, etc. Create Kotlin object ApiGetPostHelper.kt and put the below code.
package com.galleonoft.jsonparser import android.util.Log import java.io.BufferedReader import java.io.BufferedWriter import java.io.OutputStreamWriter import java.io.UnsupportedEncodingException import java.net.HttpURLConnection import java.net.URL import java.net.URLEncoder import java.util.* import javax.net.ssl.HttpsURLConnection /** * Author: JIGAR PATEL. * Tutorial_URL: https://galleonsoft.com/tutorial/ */ object ApiGetPostHelper { // Send Parameters method fun SendParams(reqURL: String, postDataParams: HashMap<String, String>?): String { val gsUrl: URL var resultString = "" try { gsUrl = URL(reqURL) val conn = gsUrl.openConnection() as HttpURLConnection conn.readTimeout = 7000 conn.connectTimeout = 7000 conn.requestMethod = "POST" conn.doInput = true conn.doOutput = true if (postDataParams != null) { // For Post encoded Parameters val os = conn.outputStream val writer = BufferedWriter(OutputStreamWriter(os, "UTF-8")) writer.write(getPostDataString(postDataParams)) writer.flush() writer.close() os.close() } else { conn.requestMethod = "GET" } val responseCode = conn.responseCode Log.i("responseCode: ", responseCode.toString() + "") if (responseCode == HttpsURLConnection.HTTP_OK) { val allTextResponse = conn.inputStream.bufferedReader().use(BufferedReader::readText) resultString = allTextResponse } else { resultString = "" } } catch (e: Exception) { resultString = "" e.printStackTrace() } return resultString } // Collect Params from HashMap and encode with url. @Throws(UnsupportedEncodingException::class) private fun getPostDataString(params: HashMap<String, String>): String { val result = StringBuilder() var first = true for ((key, value) in params) { if (first) first = false else result.append("&") result.append(URLEncoder.encode(key, "UTF-8")) result.append("=") result.append(URLEncoder.encode(value, "UTF-8")) } return result.toString() } }
5. The Finally we are implementing the activity for android JSON app. Create Kotlin class JSONParserKotlinActivity.kt and put the below code. (It’s your Activity class like MainActivity.kt)
package com.galleonoft.jsonparser import android.annotation.SuppressLint import android.content.Context import android.net.ConnectivityManager import android.os.AsyncTask import android.os.Bundle import android.support.v7.app.AppCompatActivity import android.util.Log import android.view.View import android.widget.ListView import android.widget.ProgressBar import android.widget.SimpleAdapter import android.widget.Toast import org.json.JSONArray import org.json.JSONException import org.json.JSONObject import java.util.* class JSONParserKotlinActivity : AppCompatActivity() { lateinit var Lv_developers: ListView lateinit var Pbar: ProgressBar internal var developersList = ArrayList<HashMap<String, String>>() // Checking Internet is available or not private val isNetworkConnected: Boolean get() = (getSystemService(Context.CONNECTIVITY_SERVICE) as ConnectivityManager).activeNetworkInfo != null companion object { var GetPostJSONApi = "http://apps.galleonsoft.com/api-example/json-rest-api-example.php" } // Show BackButton on Actionbar override fun onSupportNavigateUp(): Boolean { onBackPressed() return true } override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_jsonparser_kotlin) // Show BackButton and Set custom Title on Actionbar val actionBar = supportActionBar if (actionBar != null) { actionBar.title = "JSON Parser Kotlin Example" actionBar.setDisplayHomeAsUpEnabled(true) actionBar.setDisplayShowHomeEnabled(true) } // findViewById and set view id Pbar = findViewById(R.id.Pbar) Lv_developers = findViewById(R.id.Lv_developers) if (isNetworkConnected) { // Call AsyncTask for getting developer list from server (JSON Api) getDeveloper().execute() } else { Toast.makeText(applicationContext, "No Internet Connection Yet!", Toast.LENGTH_SHORT).show() } } @SuppressLint("StaticFieldLeak") inner class getDeveloper : AsyncTask<Void, Void, String>() { override fun onPreExecute() { // Show Progressbar for batter UI Pbar.visibility = View.VISIBLE } override fun doInBackground(vararg voids: Void): String { // Here is post Json api example val sendParams = HashMap<String, String>() // Send parameters and value on JSON api sendParams["Name"] = "JigarPatel" // Only Get JSON api send HashMap null see below comment example // return ApiGetPostHelper.SendParams(GetPostJSONApi, null); // Send the HttpPostRequest by HttpURLConnection and receive a Results in return string return ApiGetPostHelper.SendParams(GetPostJSONApi, sendParams) } override fun onPostExecute(results: String?) { // Hide Progressbar Pbar.visibility = View.GONE if (results != null) { // See Response in Logcat for understand JSON Results and make DeveloperList Log.i("onPostExecute: ", results) } try { // Create JSONObject from string response if your response start from Array [ then create JSONArray val rootJsonObject = JSONObject(results) val isSucess = rootJsonObject.getString("success") if (isSucess == "1") { val developerArray = rootJsonObject.getString("developers") val mJsonArray = JSONArray(developerArray) for (i in 0 until mJsonArray.length()) { // Get single JSON object node val sObject = mJsonArray.get(i).toString() val mItemObject = JSONObject(sObject) // Get String value from json object val Name = mItemObject.getString("Name") val Age = mItemObject.getString("Age") val City = mItemObject.getString("City") // hash map for single jsonObject you can create model. val mHash = HashMap<String, String>() // adding each child node to HashMap key => value/data // Now I'm adding some extra text in value mHash["Name"] = "Name: $Name" mHash["Age"] = "Age: $Age" mHash["City"] = "City: $City" // Adding HashMap pair list into developer list developersList.add(mHash) } // This is simple Adapter (android widget) for ListView val simpleAdapter = SimpleAdapter( applicationContext, developersList, R.layout.simple_listview_item, // Add String[] name same as HashMap Key arrayOf("Name", "Age", "City"), intArrayOf(R.id.tv_name, R.id.tv_age, R.id.tv_city)) Lv_developers.adapter = simpleAdapter Lv_developers.setOnItemClickListener { parent, view, position, id -> Toast.makeText(applicationContext, "Selected item is " + position, Toast.LENGTH_SHORT).show() } } else { Toast.makeText(applicationContext, "No developers found!", Toast.LENGTH_SHORT).show() } } catch (e: JSONException) { Toast.makeText(applicationContext, "Something wrong. Try Again!", Toast.LENGTH_SHORT).show() e.printStackTrace() } } } }
6. Open AndroidManifest.xml and We need to add INTERNET permission.
<uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
Then Run (Shift +F10) your android app and wait for build APK and see the screen with JSON Parser Api in android. If you have any trouble, follow github code from given link.
Conclusion: Hope you learned about Kotlin JSON parser example and its implementation for Android. Check more tutorials on GalleonSoft about Kotlin, Android Tutorials, Flutter example, Kotlin android etc.
3 Comments
I thing that in manifest file must be activity_jsonparser_kotlin, isn´t it?
sorry you could help me in the json file
Hello. I have checked your JSON Parser Tutorial.